Installation of Django
where python
set path=
E:
mkdir Django_Demo
cd Django_Demo
python -m venv MYENV
MYENV\Scripts\activate
Once the environment is activated install django.
pip install django
python –m django –-version
IT IS THE TIME TO CREATE YOUR 1st DJANGO PROJECT
django-admin startproject project_Hello
cd project_Hello
python manage.py migrate
python manage.py runserver
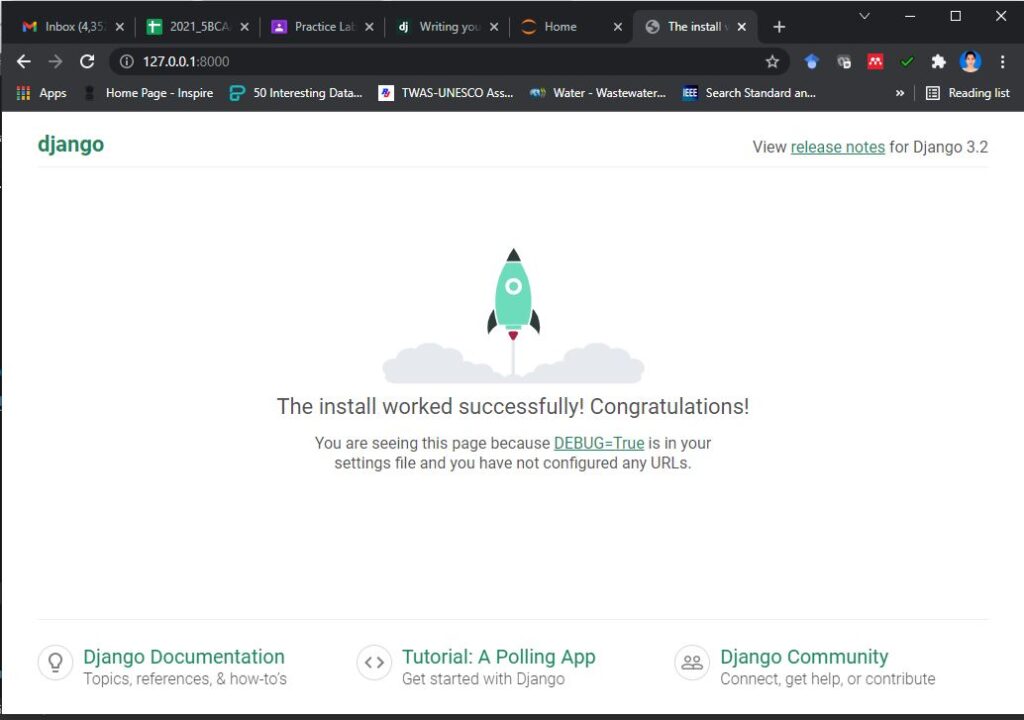
Press Ctrl+C to come back to (MYENV) E:\Django_Demo\project_Hello> path.
Inside the project directory, create a new app: Execute the following code
python manage.py startapp hello_app
Then, add hello_app to the INSTALLED_APPS section in settings.py file under the folder project_hello.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'hello_app'
]
Open views.py file which is placed inside the folder hello_app and add the following code:
from django.shortcuts import render
# Create your views here.
from django.http import HttpResponse
def function_hello_world(request):
return HttpResponse("Hello, World!")
Open urls.py file which is placed inside the folder hello_app (create urls.py file it if it doesn’t exist) and add the following code:
from django.urls import path
from .views import function_hello_world
urlpatterns = [
path("", function_hello_world), # Maps the root URL to the hello_world view
]
Open urls.py file which is placed inside the folder project_hello and add the following code:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path("",include('hello_app.urls'))
]
RUN THE DJANGO SERVER
python manage.py migrate
python manage.py runserver
Press Ctrl+C to come back to (MYENV) E:\Django_Demo\project_Hello> path.
If you have already created an environment then you have to go to the path of the folder and activate the environment to continue.
cd E:\Django_Demo
E:
MYENV\Scripts\activate
cd project_Hello
python manage.py migrate
python manage.py runserver
Login activity using Django
Activate the environment by going to the path Django_Demo folder in anaconda prompt
MYENV\Scripts\activate
Create a Django project and app for login activity:
django-admin startproject project_login
cd project_login
python manage.py startapp login_app
Add login_app
to INSTALLED_APPS
section in the file Settings.py
placed inside project_login:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'login_app'
]
Create Login and Logout Views
Open the views.py file placed inside login_app folder
and add the following code:
from django.shortcuts import render
# Create your views here.
from django.shortcuts import render, redirect
from django.contrib.auth import authenticate, login, logout
from django.contrib import messages
def login_view(request):
if request.method == "POST":
username = request.POST['username']
password = request.POST['password']
user = authenticate(request, username=username, password=password)
if user is not None:
login(request, user)
return redirect('dashboard') # Redirect after login
else:
messages.error(request, "Invalid username or password")
return render(request, 'login.html')
def logout_view(request):
logout(request)
return redirect('login')
def dashboard_view(request):
if not request.user.is_authenticated:
return redirect('login')
return render(request, 'dashboard.html', {'user': request.user})
Configure URLS
Open the urls.py file placed inside login_app folder
and add the following code:
from django.urls import path
from .views import login_view, logout_view, dashboard_view
urlpatterns = [
path('', login_view, name='login'),
path('dashboard/', dashboard_view, name='dashboard'),
path('logout/', logout_view, name='logout'),
]
Open urls.py file placed inside the folder project_login and update the content as shown below
:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('login_app.urls')),
]
Create a folder named templates inside the login_app folder and create two HTML files namely login.html and dashboard.html
Create Login and Dashboard Templates
<!DOCTYPE html>
<html>
<head>
<title>Login</title>
</head>
<body>
<h2>Login</h2>
{% if messages %}
{% for message in messages %}
<p style="color: red;">{{ message }}</p>
{% endfor %}
{% endif %}
<form method="post">
{% csrf_token %}
<label>Username:</label>
<input type="text" name="username" required><br><br>
<label>Password:</label>
<input type="password" name="password" required><br><br>
<button type="submit">Login</button>
</form>
</body>
</html>
Contents of login.html is as follows:
Content of dashboard.html file is as follows
<!DOCTYPE html>
<html>
<head>
<title>Dashboard</title>
</head>
<body>
<h2>Welcome, {{ user.username }}!</h2>
<a href="{% url 'logout' %}">Logout</a>
</body>
</html>
Create a Superuser (For Testing)
python manage.py migrate
# first migrate and then create super user
python manage.py createsuperuser
Run the Server (Give super username and password as input)
python manage.py runserver
Calculate FD returns and EMI
Step 1: Set Up Django Project
Activate the environment by going to the path Django_Demo folder in anaconda prompt
cd E:\Django_Demo
E:
MYENV\Scripts\activate
Create a Django Project
django-admin startproject project_bank
cd project_bank
Create a Django Banking App
python manage.py startapp banking_app
Register the App in settings.py
in project_bank folder
Then, add bank_app to the INSTALLED_APPS section in settings.py file under the folder project_bank.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'banking_app'
]
Step 2: Define Data Models
Open banking_app/models.py
and define models for Fixed Deposit and Loan.
Open the models.py file placed inside the banking_app and add the following code.
from django.db import models
class FixedDeposit(models.Model):
principal = models.DecimalField(max_digits=10, decimal_places=2) # Initial amount
rate_of_interest = models.FloatField() # Annual interest rate in percentage
time_period = models.IntegerField() # Time period in years
compounding_frequency = models.IntegerField(default=1) # Number of times interest is compounded per year
def calculate_fd_return(self):
"""
Formula: A = P (1 + r/n)^(nt)
Where:
A = Maturity amount
P = Principal amount
r = Annual interest rate (in decimal)
n = Compounding frequency per year
t = Time period in years
"""
P = float(self.principal)
r = float(self.rate_of_interest) / 100 # Convert percentage to decimal
n = self.compounding_frequency
t = self.time_period
A = P * (1 + r / n) ** (n * t)
return round(A, 2)
def __str__(self):
return f"FD - {self.principal} at {self.rate_of_interest}% for {self.time_period} years"
class Loan(models.Model):
loan_amount = models.DecimalField(max_digits=10, decimal_places=2) # Principal loan amount
annual_interest_rate = models.FloatField() # Annual interest rate in percentage
tenure_months = models.IntegerField() # Loan tenure in months
def calculate_emi(self):
"""
Formula: EMI = [P * r * (1+r)^n] / [(1+r)^n - 1]
Where:
P = Loan amount (principal)
r = Monthly interest rate (annual rate / 12 / 100)
n = Number of months
"""
P = float(self.loan_amount)
r = (float(self.annual_interest_rate) / 100) / 12 # Convert annual rate to monthly decimal rate
n = self.tenure_months
if r == 0: # If interest rate is 0
emi = P / n
else:
emi = (P * r * (1 + r) ** n) / ((1 + r) ** n - 1)
return round(emi, 2)
def __str__(self):
return f"Loan - {self.loan_amount} at {self.annual_interest_rate}% for {self.tenure_months} months"
class Loan(models.Model):
loan_amount = models.DecimalField(max_digits=10, decimal_places=2) # Principal loan amount
annual_interest_rate = models.FloatField() # Annual interest rate in percentage
tenure_months = models.IntegerField() # Loan tenure in months
def calculate_emi(self):
"""
Formula: EMI = [P * r * (1+r)^n] / [(1+r)^n - 1]
Where:
P = Loan amount (principal)
r = Monthly interest rate (annual rate / 12 / 100)
n = Number of months
"""
P = float(self.loan_amount)
r = (float(self.annual_interest_rate) / 100) / 12 # Convert annual rate to monthly decimal rate
n = self.tenure_months
if r == 0: # If interest rate is 0
emi = P / n
else:
emi = (P * r * (1 + r) ** n) / ((1 + r) ** n - 1)
return round(emi, 2)
def __str__(self):
return f"Loan - {self.loan_amount} at {self.annual_interest_rate}% for {self.tenure_months} months"
Apply Migrations
python manage.py makemigrations banking_app
python manage.py migrate
Step 3: Create Views for Calculation
Open views.py
placed inside the banking_app folder and define functions for FD return and EMI calculation.
from django.shortcuts import render
from .models import FixedDeposit, Loan
def fixed_deposit_view(request):
if request.method == "POST":
principal = float(request.POST.get("principal"))
rate_of_interest = float(request.POST.get("rate_of_interest"))
time_period = int(request.POST.get("time_period"))
compounding_frequency = int(request.POST.get("compounding_frequency"))
fd = FixedDeposit(
principal=principal, rate_of_interest=rate_of_interest,
time_period=time_period, compounding_frequency=compounding_frequency
)
maturity_amount = fd.calculate_fd_return()
return render(request, "fixed_deposit.html", {"maturity_amount": maturity_amount})
return render(request, "fixed_deposit.html")
def loan_emi_view(request):
if request.method == "POST":
loan_amount = float(request.POST.get("loan_amount"))
annual_interest_rate = float(request.POST.get("annual_interest_rate"))
tenure_months = int(request.POST.get("tenure_months"))
loan = Loan(
loan_amount=loan_amount, annual_interest_rate=annual_interest_rate, tenure_months=tenure_months
)
emi_amount = loan.calculate_emi()
return render(request, "loan_emi.html", {"emi_amount": emi_amount})
return render(request, "loan_emi.html")
Step 4: Define URLs
Open urls.py placed inside the folder banking_app and create URL patterns.
from django.urls import path
from .views import fixed_deposit_view, loan_emi_view
urlpatterns = [
path("fixed-deposit/", fixed_deposit_view, name="fixed_deposit"),
path("loan-emi/", loan_emi_view, name="loan_emi"),
]
Open urls.py file placed inside the folder project_bank and update the code
:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path("banking_app/", include("banking_app.urls")),
]
Step 5: Create HTML Templates
Create a folder named templates inside the folder banking_app and create two HTML files namely fixed_deposit.html and loan_emi.html
fixed_deposit.html
<form method="post">
{% csrf_token %}
Principal: <input type="number" name="principal" step="0.01" required><br>
Rate of Interest (%): <input type="number" name="rate_of_interest" step="0.01" required><br>
Time Period (Years): <input type="number" name="time_period" required><br>
Compounding Frequency: <input type="number" name="compounding_frequency" value="1" required><br>
<button type="submit">Calculate</button>
</form>
{% if maturity_amount %}
<p>Maturity Amount: {{ maturity_amount }}</p>
{% endif %}
loan_emi.html
<form method="post">
{% csrf_token %}
Loan Amount: <input type="number" name="loan_amount" step="0.01" required><br>
Annual Interest Rate (%): <input type="number" name="annual_interest_rate" step="0.01" required><br>
Tenure (Months): <input type="number" name="tenure_months" required><br>
<button type="submit">Calculate</button>
</form>
{% if emi_amount %}
<p>Monthly EMI: {{ emi_amount }}</p>
{% endif %}
Step 6: Run the Project
python manage.py runserver
Use the following URLs
http://127.0.0.1:8000/banking_app/fixed-deposit/
http://127.0.0.1:8000/banking_app/loan-emi/